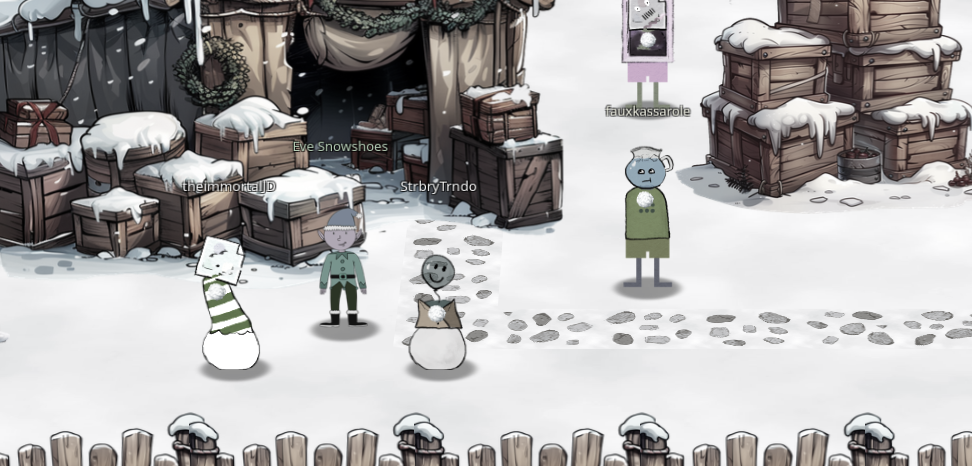
Starting off Act 2 we have a returning challenge in Android App Analysis. Talking to Eve Snowshoes, she will tell us that she’s been working on a new app for the naughty and nice list and has a debug and a release version. Each version is missing a child on the list and we need to identify who that is
Silver Medal
As usual we’ll start with solving the silver medal. This is done on the debug APK which can just be loaded into jadx. Once loaded, we can start parsing through the source code
The first thing that jumped out to me was in com.northpole.santaswipe.DatabaseHelper
. It would make sense for their to be a database associated with the children on the list and checking the Class I could see all the database creation statements. From here I installed the apk onto an android device I had and simply compared the two lists of names to find the missing one, 'Ellie, Alabama, USA'
. Entering Ellie grants the silver medal.
Gold Medal
For the Gold medal the initial strategy was still the same. I loaded the .aab into jadx and went to check the database Class but this time the values were no longer in plain text. The first line that jumped out to me was at line 39. This is a sql command that first calls a function decryptData
against a long string. Checking decryptData
we can see the parameters that were used for encryption. It’s an AES GCM cipher with no padding. The string itself also appears to be in base64 format.
Since the cipher is aes GCM we need to find the key and iv values. In Android apps these are stored in res/values/strings.xml
.
To find this file I followed the hint from the elf to turn the aab into an apk. This can be done with bundletool
and the commands below
java -jar bundletool-all-1.17.2.jar build-apks --bundle=./SantaSwipeSecure.
aab --output santaswipesecure.apks --mode=universalmv santaswipesecure.apks santaswipesecure.zip
unzip santaswipesecure.zip
This apk can then be decompiled with apktool
using apktool d universal.apk
and from here we can navigate to universal/res/values/strings.xml
Down towards the bottom of the file we should find both values:
- IV: Q2hlY2tNYXRlcml4
- ek: rmDJ1wJ7ZtKy3lkLs6X9bZ2Jvpt6jL6YWiDsXtgjkXw=
Now with all of this put together we can write a python script to decode and print the data.
from cryptography.hazmat.primitives.ciphers import Cipher, algorithms, modes
from cryptography.hazmat.backends import default_backend
import base64
# Provided values
encrypted_data = "IVrt+9Zct4oUePZeQqFwyhBix8cSCIxtsa+lJZkMNpNFBgoHeJlwp73l2oyEh1Y6AfqnfH7gcU9Yfov6u70cUA2/OwcxVt7Ubdn0UD2kImNsclEQ9M8PpnevBX3mXlW2QnH8+Q+SC7JaMUc9CIvxB2HYQG2JujQf6skpVaPAKGxfLqDj+2UyTAVLoeUlQjc18swZVtTQO7Zwe6sTCYlrw7GpFXCAuI6Ex29gfeVIeB7pK7M4kZGy3OIaFxfTdevCoTMwkoPvJuRupA6ybp36vmLLMXaAWsrDHRUbKfE6UKvGoC9d5vqmKeIO9elASuagxjBJ"
key_b64 = "rmDJ1wJ7ZtKy3lkLs6X9bZ2Jvpt6jL6YWiDsXtgjkXw="
iv_b64 = "Q2hlY2tNYXRlcml4"
# Decode the base64-encoded data
ciphertext = base64.b64decode(encrypted_data)
key = base64.b64decode(key_b64)
iv = base64.b64decode(iv_b64)
# Prepare for AES-GCM decryption
# We assume that the ciphertext includes the tag at the end (common in AES-GCM)
tag = ciphertext[-16:]
ciphertext_without_tag = ciphertext[:-16]
# Initialize the AES-GCM cipher
cipher = Cipher(algorithms.AES(key), modes.GCM(iv, tag), backend=default_backend())
decryptor = cipher.decryptor()
# Decrypt the ciphertext
plaintext = decryptor.update(ciphertext_without_tag) + decryptor.finalize()
# Output the plaintext
print("Decrypted message:", plaintext.decode('utf-8'))
This returns Joshua, Birmingham, United Kingdom
and entering in Joshua
grants the gold medal.
Leave a Reply